How to create lyrics post generator in php
Author: DreamPirates | Last Updated : 0000-00-00 00:00:00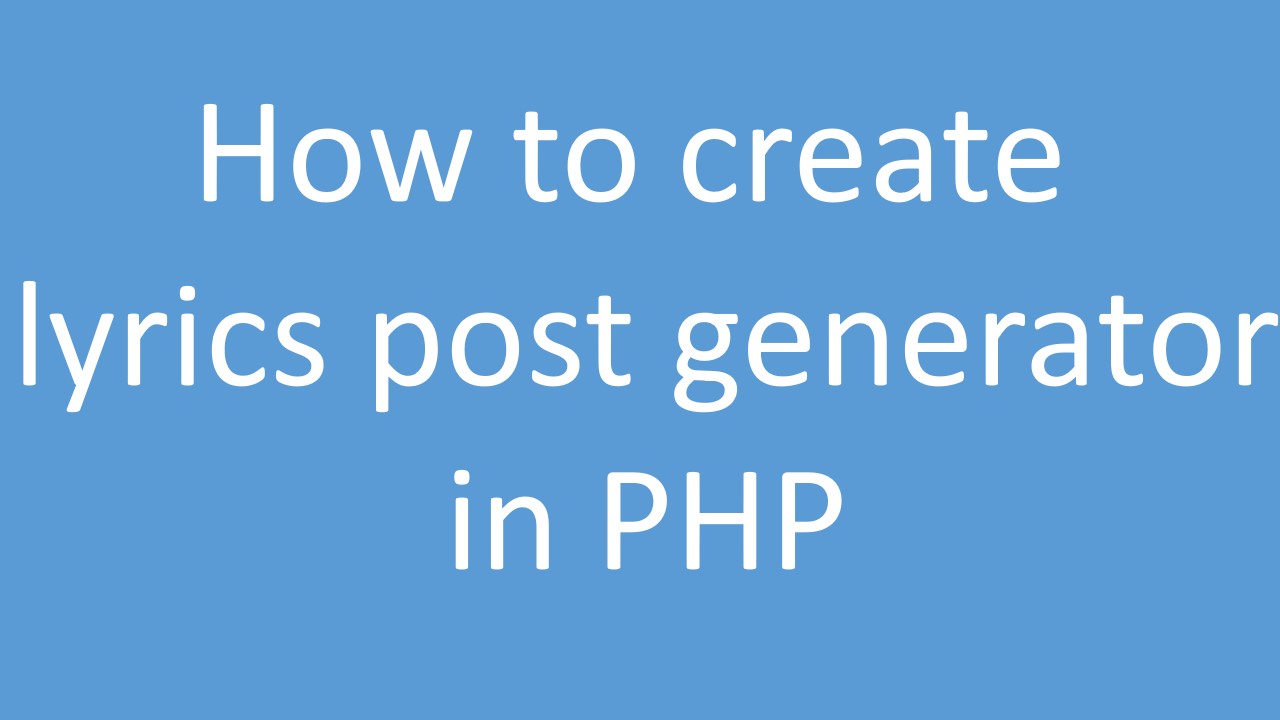
Step : 1
Create page index.php . Now What data will need to create lyrics post
- youtube url
- song title
- singer
- composer
- music
- lyrics writer
- Lyrics
We will get this data using form .
<form method="post" action="output">
<table>
<tbody><tr><td>Youtube Video URL </td><td><input type="text" name="url" placeholder="Enter Video URL" required=""></td></tr>
<tr><td>Song Title </td><td><input type="text" name="title" placeholder="Enter Song Title" required=""></td></tr>
<tr><td>Singer</td><td><input type="text" name="singer" placeholder="Enter Singer name" required=""></td></tr>
<tr><td>Composer</td><td><input type="text" name="composer" placeholder="Enter Composer name"></td></tr>
<tr><td>Music</td><td><input type="text" name="music" placeholder="Enter"></td></tr>
<tr><td>Lyrics Writer</td><td><input type="text" name="writer" placeholder="Enter writer name"></td></tr>
<tr><td>Full Lyrics</td><td><textarea name="lyrics" class="" style="width: 400px; height: 200px;" white-space:="" pre-wrap;="" required=""></textarea></td></tr>
<tr><td></td><td><input type="submit" name="submit" class="button" value="GENERATE MY POST"></td></tr>
</tbody></table>
</form>
save this code in index.php file In this we are using POST method (method="post") to send data to OUTPUT (acton="output")page.
STEP 2 :
Create output.php file in same directory
in this file we will get data from index file using post
$url = $_POST['url'];
here we will get data from <input type="text" name="url" placeholder="Enter Video URL" required="">
do same for all data .
$url = $_POST['url'];
$title = $_POST['title'];
$singer = $_POST["singer"];
$composer = $_POST['composer'];
$music = $_POST['music'];
$writer = $_POST['writer'];
$ly = $_POST['lyrics'];
Step 3 :
Now We need to get youtude id from url
Thre are 2 type of youtue video url
- https://www.youtube.com/watch?v=w13AKEugxoY
- https://youtu.be/w13AKEugxoY
We only need id so we will filter url using str_replace method
$url2 = str_replace("https://www.youtube.com/watch?v=","",$url1); //removing https://www.youtube.com/watch?v= from url
$ytid = str_replace("https://youtu.be/","",$url2); //removing https://youtu.be/ from URL
Final Rresult will be $ytid = ''w13AKEugxoY"
Step 4 : Now we will process lyrics data to keep space and line as it is.
$lyrics = nl2br($ly);
Now code will look like this
<?php
$url = $_POST['url'];
$url1 = str_replace(" ","",$url);
$url2 = str_replace("https://www.youtube.com/watch?v=","",$url1);
$ytid = str_replace("https://youtu.be/","",$url2);
$title = $_POST['title'];
$singer = $_POST["singer"];
$composer = $_POST['composer'];
$music = $_POST['music'];
$writer = $_POST['writer'];
$ly = $_POST['lyrics'];
$lyrics = nl2br($ly);
?>
STEP 5 : Now We need to print output.Make smple lyrics post and replace changing part with php code and keep comman part
https://img.youtube.com/vi/w13AKEugxoY/maxresdefault.jpg this is same for youtube video hd thumbnail.
<textarea style="width:100%%; height: 300px;">
<div>
<h2><?php echo $title." - ".$singer;?> Lyrics</h2>
<hr>
<img src='https://img.youtube.com/vi/<?php echo $ytid;?>/maxresdefault.jpg' alt="<?php echo $title;?>" width="100%%"/>
<hr>
<style>
table{
border-spacing: 0px;
border: solid 1px black;
padding:10px;
}
tr,td{
border-spacing: 0px;
border: solid 1px black;
padding:10px;
}
</style>
<table>
<tr><td>Singer</td> <td><?php echo $singer;?></td></tr>
<tr><td>Composer</td> <td><b><?php echo $composer;?></b></td></tr>
<tr><td>Music</td> <td> <b><?php echo $music;?></b></td></tr>
<tr><td>Song Writer</td><td><b><?php echo $writer;?></b></td></tr>
</table>
<hr>
<h3>Lyrics</h3>
<divstyle='text-align: center;'>
<?php echo $lyrics;?>
</div>
<br>
<br>
<h2><?php echo $title;?> Watch Video</h2>
<iframe frameborder="0" scrolling="no" marginheight="0" marginwidth="0" width="100%%" height="360" type="text/html" src="https://www.youtube.com/embed/<?php echo $ytid;?>?autoplay=0&origin=https://www.dreampirates.in"></iframe>
</div>
</textarea>
save this file as output.php file now test the code.
final code for output.php
<?php
$url = $_POST['url'];
$url1 = str_replace(" ","",$url);
$url2 = str_replace("https://www.youtube.com/watch?v=","",$url1);
$ytid = str_replace("https://youtu.be/","",$url2);
$title = $_POST['title'];
$singer = $_POST["singer"];
$composer = $_POST['composer'];
$music = $_POST['music'];
$writer = $_POST['writer'];
$ly = $_POST['lyrics'];
$lyrics = nl2br($ly);
?><textarea style="width:100%%; height: 300px;">
<div>
<h2><?php echo $title." - ".$singer;?> Lyrics</h2>
<hr>
<img src='https://img.youtube.com/vi/<?php echo $ytid;?>/maxresdefault.jpg' alt="<?php echo $title;?>" width="100%%"/>
<hr>
<style>
table{
border-spacing: 0px;
border: solid 1px black;
padding:10px;
}
tr,td{
border-spacing: 0px;
border: solid 1px black;
padding:10px;
}
</style>
<table>
<tr><td>Singer</td> <td><?php echo $singer;?></td></tr>
<tr><td>Composer</td> <td><b><?php echo $composer;?></b></td></tr>
<tr><td>Music</td> <td> <b><?php echo $music;?></b></td></tr>
<tr><td>Song Writer</td><td><b><?php echo $writer;?></b></td></tr>
</table>
<hr>
<h3>Lyrics</h3>
<divstyle='text-align: center;'>
<?php echo $lyrics;?>
</div>
<br>
<br>
<h2><?php echo $title;?> Watch Video</h2>
<iframe frameborder="0" scrolling="no" marginheight="0" marginwidth="0" width="100%%" height="360" type="text/html" src="https://www.youtube.com/embed/<?php echo $ytid;?>?autoplay=0&origin=https://www.dreampirates.in"></iframe>
</div>
</textarea>
Conclution : We successfully creatated Lyrics post generator . :)
Tag : howto
Relative Posts

How To Run Multiple Monitor On Single CPU
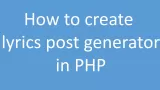